In this 2nd part I made a more complex example to be more practical in real life, and created a web app that is gets its users from db and stores the clients, and tokens in db instead of memory so it's more scalable.
features:
1- users & roles in database
2- oauth related info stored in db (tokens, clients, approvals, etc..)
3- two APIs : a- cats apis, b- dogs api; each one of these can be accessed only if the access token has a proper scope (cats scope and dogs scope)
the app uses h2 in memory database so that it be self contained and no external setup is required
and here is the source code :
https://github.com/blabadi/oauth2lw-parent/tree/master/oauth2lw-client-jdbc
show case:
1- get access token to with scope cats:
a- get the auth code :
go to : localhost:8080/oauth/authorize?response_type=code&client_id=cats-client&redirect_uri=http://localhostcats/redirect
2- login with a user
3- approve the scopes :
4- get the authorization code
5- exchange the code for a token
6- access cats api:
7- try the dogs api
try to get a valid token to access the dogs api by yourself should be similar to the cats process..
get familiar with the source code, it's only a step up and we only used the classes from the oauth2lw-core we added the jdbc datasource and the token store and passed these to our configures.
Sunday, January 8, 2017
Saturday, January 7, 2017
Spring Security OAuth2 + Spring Boot, minimalist working, extendable configuration - Part 1
Hi
been a while again..
today i'm back to write on how to write the simplest possible spring boot app protected with spring security oauth2, but i'll also make the project as a library jar that can be reused for any new projects to kick off faster.
and it will be a simple ready to override functions that will let you focus on configuring only things that you need to worry about without having to worry about annotations or other spring security stuff.
Overview
Spring security oauth 2 has three main components :
a- Authorization server: to handle tokens/authroization codes and user approve/deny
b- Resource server: protects the actual apis that we want to be protected by oauth2
c- spring web security configs to manage users authentication
and as simple as it sounds to get these three in one simple hello world example required hours and hours of searching and debugging dont ask me why..
Pre Requests :
1- you know spring security & spring web security concepts and how to configure it like basic auth or form login
so without further due here are the three classes you will need to get oauth2 up and running :
1- Spring security:
https://github.com/blabadi/oauth2lw-parent/blob/master/oauth2lw-core/src/main/java/com/bashar/oauth2lw/core/SecurityConfig.java
this class extends the Spring web security configurer to allow us to set how we want to protect our authorization server, and to set up our users (Resource owners) store. here it's in memory but in your application you will need jdbc so you can just extend and override this.
by default this protects /oauth/authroize api with the default spring web security login form
when you need to override that form you should override this method :
protected void configure(HttpSecurity http)
and set the url of the login page of your choice using the usual spring web security configs.
2- Authroization server:
https://github.com/blabadi/oauth2lw-parent/blob/master/oauth2lw-core/src/main/java/com/bashar/oauth2lw/core/AuthorizationServer.java
here this configuration server has two main parts :
A- configure(ClientDetailsServiceConfigurer clients)
this method configures the clients of our oauth2 resource, and clients in 90% of the cases in the world of oauth2 represents the 3rd party apps that want to access the resource owner data on his behalf (as example of that is when you allow a web or mobile application to access your instagram photos or facebook friends list)
B- configure(AuthorizationServerEndpointsConfigurer endpoints)
this method let you configure the oauth2 endpoints settings as: where to store the tokens, codes, and many other customizations that are usually not needed (the most important one is setting the token store)
3- Resource Server.java
here we can configure 2 important things
https://github.com/blabadi/oauth2lw-parent/blob/master/oauth2lw-core/src/main/java/com/bashar/oauth2lw/core/ResourceServer.java
1- configure(ResourceServerSecurityConfigurer resources)
this can allow us to configure the id of this resource server (becomes handy if we have multiple resource servers and we have access rules for different clients on each of them) and the more important config is the token store, i.e. where to verify the tokens that we receive in the requests and make sure they are authentic and created by our Authorization server.
2- configure(HttpSecurity http)
this is important and allows us to configure the urls that we want to protect under the oauth2 protocl in this resource, anything we specify here will require a valid token that can access this resource propery and in my default configuration i protected any api under the path /protected to be fully authenticated, now this is app specific configs because each app has its own apis and roles and security rules, so make sure you understand how to use the HttpSecurity builder
it worths mentioning the oauth2 spring libraries provide some exprissions like hasScope() and other security functions related to oauth2 security.
now how to use these classes?
you just have to add this jar in your deps in maven or gradle or whatever and import these 3 classes to your spring configurations, I have made an example in this client project:
https://github.com/blabadi/oauth2lw-parent/blob/master/oauth2lw-client/src/main/java/com/bashar/oauth2lw/client/Oauth2lwClientApplication.java
this is simple spring boot application and in it, just for practicing i overrode the method that configures clients in Authorization server and added my own client.. this can be converted to jdbc or whatever you want
and for the other 2 classes I haven't changed anything so I just imported them as is, if I haven't changed anything in Authorization server I would have also just imported it, but it will be scanned automatically since we extended it and annotated it with @Configration
and when we run the application and as for a protected (although non existing) url like :
so to get a token there are many flows but the more complex and more typical that we would use oauth2 for is authorization code flow, so to obtaina token go to the browser and type in :
http://localhost:8080/oauth/authorize?response_type=code&client_id=trusted1&redirect_uri=http://localhost:9090/redirect
this will redirect us to login page where this is similar to when you are redirected to facebook by a third party app to login and approve its permissions to get some of your data
so we login with our dummy in memory user setup in the spring security configs:
then you get the approval of scopes page
Simple and clean and ready to be used out of the box just get the jar of the oauth2lw-core and drop it in your project and you will have oauth2 security ready.
note that the core lib pom already have the required deps:
org.springframework.cloud
spring-cloud-starter-oauth2
so no need for you to worry about it..
been a while again..
today i'm back to write on how to write the simplest possible spring boot app protected with spring security oauth2, but i'll also make the project as a library jar that can be reused for any new projects to kick off faster.
and it will be a simple ready to override functions that will let you focus on configuring only things that you need to worry about without having to worry about annotations or other spring security stuff.
Overview
Spring security oauth 2 has three main components :
a- Authorization server: to handle tokens/authroization codes and user approve/deny
b- Resource server: protects the actual apis that we want to be protected by oauth2
c- spring web security configs to manage users authentication
and as simple as it sounds to get these three in one simple hello world example required hours and hours of searching and debugging dont ask me why..
Pre Requests :
1- you know spring security & spring web security concepts and how to configure it like basic auth or form login
so without further due here are the three classes you will need to get oauth2 up and running :
1- Spring security:
https://github.com/blabadi/oauth2lw-parent/blob/master/oauth2lw-core/src/main/java/com/bashar/oauth2lw/core/SecurityConfig.java
this class extends the Spring web security configurer to allow us to set how we want to protect our authorization server, and to set up our users (Resource owners) store. here it's in memory but in your application you will need jdbc so you can just extend and override this.
by default this protects /oauth/authroize api with the default spring web security login form
when you need to override that form you should override this method :
protected void configure(HttpSecurity http)
and set the url of the login page of your choice using the usual spring web security configs.
2- Authroization server:
https://github.com/blabadi/oauth2lw-parent/blob/master/oauth2lw-core/src/main/java/com/bashar/oauth2lw/core/AuthorizationServer.java
here this configuration server has two main parts :
A- configure(ClientDetailsServiceConfigurer clients)
this method configures the clients of our oauth2 resource, and clients in 90% of the cases in the world of oauth2 represents the 3rd party apps that want to access the resource owner data on his behalf (as example of that is when you allow a web or mobile application to access your instagram photos or facebook friends list)
B- configure(AuthorizationServerEndpointsConfigurer endpoints)
this method let you configure the oauth2 endpoints settings as: where to store the tokens, codes, and many other customizations that are usually not needed (the most important one is setting the token store)
3- Resource Server.java
here we can configure 2 important things
https://github.com/blabadi/oauth2lw-parent/blob/master/oauth2lw-core/src/main/java/com/bashar/oauth2lw/core/ResourceServer.java
1- configure(ResourceServerSecurityConfigurer resources)
this can allow us to configure the id of this resource server (becomes handy if we have multiple resource servers and we have access rules for different clients on each of them) and the more important config is the token store, i.e. where to verify the tokens that we receive in the requests and make sure they are authentic and created by our Authorization server.
2- configure(HttpSecurity http)
this is important and allows us to configure the urls that we want to protect under the oauth2 protocl in this resource, anything we specify here will require a valid token that can access this resource propery and in my default configuration i protected any api under the path /protected to be fully authenticated, now this is app specific configs because each app has its own apis and roles and security rules, so make sure you understand how to use the HttpSecurity builder
it worths mentioning the oauth2 spring libraries provide some exprissions like hasScope() and other security functions related to oauth2 security.
now how to use these classes?
you just have to add this jar in your deps in maven or gradle or whatever and import these 3 classes to your spring configurations, I have made an example in this client project:
https://github.com/blabadi/oauth2lw-parent/blob/master/oauth2lw-client/src/main/java/com/bashar/oauth2lw/client/Oauth2lwClientApplication.java
this is simple spring boot application and in it, just for practicing i overrode the method that configures clients in Authorization server and added my own client.. this can be converted to jdbc or whatever you want
and for the other 2 classes I haven't changed anything so I just imported them as is, if I haven't changed anything in Authorization server I would have also just imported it, but it will be scanned automatically since we extended it and annotated it with @Configration
and when we run the application and as for a protected (although non existing) url like :
so to get a token there are many flows but the more complex and more typical that we would use oauth2 for is authorization code flow, so to obtaina token go to the browser and type in :
http://localhost:8080/oauth/authorize?response_type=code&client_id=trusted1&redirect_uri=http://localhost:9090/redirect
this will redirect us to login page where this is similar to when you are redirected to facebook by a third party app to login and approve its permissions to get some of your data
so we login with our dummy in memory user setup in the spring security configs:
then you get the approval of scopes page
and if the user approves, then the server will issue an authorization code and return it to the 3rd party app on the redirect uri provided
the 3rd party server now should exchange that code with our server to an access token
after getting the token it can now use it to access the protected resoruce that we couldn't access before
Simple and clean and ready to be used out of the box just get the jar of the oauth2lw-core and drop it in your project and you will have oauth2 security ready.
note that the core lib pom already have the required deps:
so no need for you to worry about it..
Subscribe to:
Posts (Atom)
Istio —simple fast way to start
istio archeticture (source istio.io) I would like to share with you a sample repo to start and help you continue your jou...
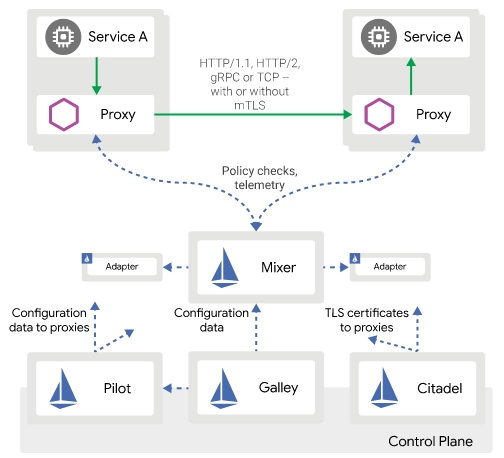
-
So RecyclerView was introduced to replace List view and it's optimized to reuse existing views and so it's faster and more efficient...
-
I worked before on learning angular 2+, and made a sample functioning app that tracks calories & macros, called it ...
-
In the previous part we finished the dashboard read functionality, now we want to add the skeleton for other pages: - Login In this p...