In this post I'll explain the required work to create a rest API utilizing both spring and hibernate version 4, and the configuration will be using java configuration classes not XML.
I'll use gradle to build and for dependency management, it's way easier than maven and keeps you focused on the application, if you are not familiar with gradle and interested in it see my previous post about it.
The first part which is dependency management is covered in gradle post mentioned above.
I'll skip to explain each tier of the project and its configurations:
As you can see we have 4 tiers:
1) DAO tier / data tier
In this tier we configure the datasource and hibernate, I used HSQL in memory db it can be easily substituted with other db engine providing the right dependencies
The DaoConfig defines the data source, transaction manager, session factory and hibernate properties
The most important part is the annotations :
1) @Configuration : to tell spring that this is a configuration class
2) @PropertySource("classpath:dao.properties") : is to load the properites file for this configuration
3) @ComponentScan({ "com.blabadi.gradle.dao" }) : is to tell spring container to scan the specific packages to look for beans, like Dao classes and entities
4) @EnableTransactionManagement : is similar to xml configuration <tx:annotation-driven>
responsible for registering the necessary Spring components that power
annotation-driven transaction management (to be able to use @Transactional).
The rest is the person DO and the person DAO :
2) Business logic tier
configuration here is simple, and the person service is utilizing the @Transnational annotation
3) Rest tier
this is where the web app configuration is, and exposes the Person APIs
The WebConfig.java contains the spring web configuration for the rest project, it uses the @EnableWebMvc to configure the spring dispatcher servlet.
The WebAppInit.java is actually the replacement for web.xml in the java servlet 3 standard it looks for a class the implements WebApplicationInitializer interface as replacement for the web.xml
Note that I had to add the dependency for servlet-api-3.0.1 to my gradle as compile time dependency but that's not the best way, because it will be provided by the application server.
Wednesday, September 24, 2014
Sunday, September 21, 2014
Gradle : Next-gen build tool introduction
I've been recently reading about maven 3 and from a page to another I read a bit about gradle, and got interested in it, so I want to try it now and see how it feels and works in practice
so to get started you need to download gradle from here: http://www.gradle.org/downloads
then add the path to gradle bin directory to the PATH system variable and go to CMD/shell then run:
> gradle
if it worked and you got build successful let's move to setup our project structure.
a word about gradle first, is that it promises to keep the great conventions Maven provided but with the flexibility ANT had since Maven can give you a hard time if you need to customize something.
it's a convention over configuration, yet flexible.
so back to the track, what I want to do in this project is to setup a full spring-mvc rest API server, with multiple tiers (projects) that contains DAOs, Business Entities, Services, and REST tier.
o---[ REST ] -- uses --> [Services] -- uses --> [DAOs] --- > (DB)
| | |
[ Business Entitiess ]
this is a simple enterprise architecture to provide loosely coupled tiers, to simulate a real project structure to test what gradle has to offer here.
In maven I would go and create a parent project then add these projects as modules, but when I need to build a project I'll have to build every thing which takes a lot of time.
ok so in gradle we have 3 options to structure our build files [build.gradle]
1) Individual : each sub project has it's own build file
2) Unified : one build file in the master project to build all sub projects
3) Hybrid: a build file in the master project that contains common build configurations and build files in each sub project that are specific for each build file
the Hybrid in my opinion gives you the best of the two worlds so i'll use it here, the other two approaches will implicitly be implemented.
A. Setting up the project structure
Now the directory structure of our project, i'll go to file system and create the following JSON tree:
master-proj {
build.gradle,
settings.gradle,
dao : { src :{ main : { java : {} , resources : {} } , test :{}} , build.gradle },
be : { source :{ code : { java : {} , res : {} } , test :{} }, build.gradle },
bl : { src :{ main : { java : {} , resources : {} } , test :{}} , build.gradle },
rest :{ src :{ main : { java : {} , resources : {} } , test :{}} , build.gradle }
}
note: i'll be using git also in this tutorial to keep a reference for the code.
if you notice the 'be' src structure is different to test how easy it is to changed default project layout (src/main/java and src/main/resources) that follows maven conventions.
after creating this structure the content of build.gradle in the master project for now will only contain:
the contents of settings.gradle will be :
these are the names of the directories that contain the sub projects
navigate in cmd to master-proj directory and and run :
what you will get is build directories inside each project like this:
Now let's go to be/build.gradle and add the following segment :
add the following class in be/source/code/java folder (don't forget to add the directories for the package) :
navigate to master-proj/be and run :
open the compiler jar in lib to see it's contents:
so now we have something we can work with as start point.
now we want to import this to eclipse but we need to add eclipse config files ( .classpath, .project, .settings) gradle has a plugin for that and we can include it like the java plugin under our master project build file :
now all your projects can be imported to eclipse to work with easier.
let's go ahead and do that:
it also figured out about our custom source directory in project 'be'
B. Dependency management
in our work we usually need the following types of dependencies:
1- 3rd party dependencies in runtime (spring jars)
2- 3rd party dependencies in compile time only ( javax.servlets )
3- 3rd party testing dependencies (junit)
4- our own frameworks jars / generated web services client jars
5- projects dependencies
some of these are on maven repositories and others aren't, gradle allows us to do everything from importing a jar from file system like ant or use central maven repo or use our own maven repo if we have one, to test this let's plan to add the following dependencies:
for DAO project it will depend on :
- spring for Dependency injection
- hibernate for persistence
- business entities project
for BL :
- spring for Dependency injection
- our own utility library [local jar]
- business entities project
- Junit4 for testing
for REST :
- spring for Dependency injection & spring web
- BL project
- BE project
BE project won't have any dependencies for now.
easiest way to get spring dependencies is to use maven central repo, and since spring core is common between more than one project let's configure this in one place.
In the master project build file i'll add this :
this tells gradle to user maven central repo to download the spring context jar (and it's dependencies)
and for each of the three projects add the spring-context jar as a compile time dependency
now run gradle eclipse in the master project, it will download the spring jars then update the class path for the three projects to reference the spring-context jar from the local
for the REST project it needs spring-webmvc.jar to expose our rest api's so in rest/build.gradle
add this :
and run gradle eclipse to update classpath file
for the dao we will need hibernate jars so add this to its build.gradle:
now let's convert REST project to a WAR instead of JAR, to do that add the following plugin at the top of its build file :
and add the following dependency :
this is how we add a dependency between the projects
now also add the following to complete the dependency between our projects :
in master-project/build.gradle :
and in the bl/build.gradle:
assume we want our library in the root project in folder called libs, for example I have put xpp3.jar in that folder and I want to reference it inside my projects
1- add flat directory repository :
repositories {
mavenCentral( )
flatDir {
dirs "$rootDir/libs"
}
}
$rootDir is a DSL variable that points to the root project base directory.
and in the dependencies add :
compile 'xpp3:xpp3:1.1.4'
it will now be resolved to the libs directory without the need to complex configuration like maven.
another way to do this is to use the files( ) method without declaring a flatDir repository:
compile files('../libs/commons-fileupload-1.3.1.jar') //[assuming we are in a subproject depedency ]
see the master-proj build.gradle :
ok now the final step is prepare our application for deployment, I have took some time to add the required spring configurations and wire everything together I added few dependencies for junit in the master project build.gradle:
to deploy the project run :
gradle build and you will get a war from the rest project, drop that in your server and you're good to go
visit the final project setup here on git hub:
https://github.com/blabadi/hello-world/tree/dev/test-gradle/master-proj
Summary :
Gradle is simple, flexible and really powerful, the only thing took time is boilerplate required for the initial structure which I did manually on purpose to understand the very basics of gradle.
Gradle yet has more interesting features, and what I only covered is mainly the java plugin and maven in gradle, the possibilities are endless, since you are using groovy scripting for the build domain (DSL) which provides easy to change conventions.
refs :
1- http://www.gradle.org/docs/current/userguide/
2- http://spring.io/guides/gs/gradle/
so to get started you need to download gradle from here: http://www.gradle.org/downloads
then add the path to gradle bin directory to the PATH system variable and go to CMD/shell then run:
> gradle
if it worked and you got build successful let's move to setup our project structure.
a word about gradle first, is that it promises to keep the great conventions Maven provided but with the flexibility ANT had since Maven can give you a hard time if you need to customize something.
it's a convention over configuration, yet flexible.
so back to the track, what I want to do in this project is to setup a full spring-mvc rest API server, with multiple tiers (projects) that contains DAOs, Business Entities, Services, and REST tier.
o---[ REST ] -- uses --> [Services] -- uses --> [DAOs] --- > (DB)
| | |
[ Business Entitiess ]
this is a simple enterprise architecture to provide loosely coupled tiers, to simulate a real project structure to test what gradle has to offer here.
In maven I would go and create a parent project then add these projects as modules, but when I need to build a project I'll have to build every thing which takes a lot of time.
ok so in gradle we have 3 options to structure our build files [build.gradle]
1) Individual : each sub project has it's own build file
2) Unified : one build file in the master project to build all sub projects
3) Hybrid: a build file in the master project that contains common build configurations and build files in each sub project that are specific for each build file
the Hybrid in my opinion gives you the best of the two worlds so i'll use it here, the other two approaches will implicitly be implemented.
A. Setting up the project structure
Now the directory structure of our project, i'll go to file system and create the following JSON tree:
master-proj {
build.gradle,
settings.gradle,
dao : { src :{ main : { java : {} , resources : {} } , test :{}} , build.gradle },
be : { source :{ code : { java : {} , res : {} } , test :{} }, build.gradle },
bl : { src :{ main : { java : {} , resources : {} } , test :{}} , build.gradle },
rest :{ src :{ main : { java : {} , resources : {} } , test :{}} , build.gradle }
}
note: i'll be using git also in this tutorial to keep a reference for the code.
if you notice the 'be' src structure is different to test how easy it is to changed default project layout (src/main/java and src/main/resources) that follows maven conventions.
after creating this structure the content of build.gradle in the master project for now will only contain:
allProjects {this tells gradle that all sub projects will use the java plugin without explicitly saying that in the sub projects build files
apply plugin: 'java'
}
the contents of settings.gradle will be :
include 'dao', 'be', 'bl', 'rest'
these are the names of the directories that contain the sub projects
navigate in cmd to master-proj directory and and run :
> gradle build
what you will get is build directories inside each project like this:
Now let's go to be/build.gradle and add the following segment :
apply plugin: 'java'This will tell gradle java plugin where to find our source code, i'll leave test directory for later.
sourceSets {
main {
java {
srcDir 'source/code/java'
}
resources {
srcDir 'source/code/res'
}
}
}
add the following class in be/source/code/java folder (don't forget to add the directories for the package) :
package com.blabadi.gradle.be;and in the be/source/code/res folder add an empty file call it : be.properties
public class Person {
}
navigate to master-proj/be and run :
>gradle buildgo to master-proj\be\build, you will find more directories : (classes, dependency-cahce, resources)
open the compiler jar in lib to see it's contents:
so now we have something we can work with as start point.
now we want to import this to eclipse but we need to add eclipse config files ( .classpath, .project, .settings) gradle has a plugin for that and we can include it like the java plugin under our master project build file :
allprojects {
apply plugin: 'java'
apply plugin: 'eclipse'
}
now while you are in in master-proj dir , run :
gradle eclipse
now all your projects can be imported to eclipse to work with easier.
let's go ahead and do that:
it also figured out about our custom source directory in project 'be'
B. Dependency management
in our work we usually need the following types of dependencies:
1- 3rd party dependencies in runtime (spring jars)
2- 3rd party dependencies in compile time only ( javax.servlets )
3- 3rd party testing dependencies (junit)
4- our own frameworks jars / generated web services client jars
5- projects dependencies
some of these are on maven repositories and others aren't, gradle allows us to do everything from importing a jar from file system like ant or use central maven repo or use our own maven repo if we have one, to test this let's plan to add the following dependencies:
for DAO project it will depend on :
- spring for Dependency injection
- hibernate for persistence
- business entities project
for BL :
- spring for Dependency injection
- our own utility library [local jar]
- business entities project
- Junit4 for testing
for REST :
- spring for Dependency injection & spring web
- BL project
- BE project
BE project won't have any dependencies for now.
easiest way to get spring dependencies is to use maven central repo, and since spring core is common between more than one project let's configure this in one place.
In the master project build file i'll add this :
allprojects {
apply plugin: 'java'
apply plugin: 'eclipse'
repositories { mavenCentral() }
}
[':bl', ':dao', ':rest'].each {
pn -> project(pn) { dependencies
{ compile 'org.springframework:spring-context:4.1.0.RELEASE' }
}
}
this tells gradle to user maven central repo to download the spring context jar (and it's dependencies)
and for each of the three projects add the spring-context jar as a compile time dependency
now run gradle eclipse in the master project, it will download the spring jars then update the class path for the three projects to reference the spring-context jar from the local
for the REST project it needs spring-webmvc.jar to expose our rest api's so in rest/build.gradle
add this :
dependencies {
compile 'org.springframework:spring-webmvc:4.1.0.RELEASE'
}
and run gradle eclipse to update classpath file
for the dao we will need hibernate jars so add this to its build.gradle:
dependencies {
compile 'org.hibernate:hibernate-core:4.3.6.Final'
}
now let's convert REST project to a WAR instead of JAR, to do that add the following plugin at the top of its build file :
apply plugin: 'war'
and add the following dependency :
dependencies {
compile 'org.springframework:spring-webmvc:4.1.0.RELEASE'
compile project(':bl')}
this is how we add a dependency between the projects
now also add the following to complete the dependency between our projects :
in master-project/build.gradle :
[':bl', ':dao', ':rest'].each { pn ->
project(pn) {
dependencies {
compile 'org.springframework:spring-context:4.1.0.RELEASE'
compile project(':be') }
}
}
and in the bl/build.gradle:
dependencies {to add a local jar (not 3rd party and not in a Maven repo) we can do the following:
compile project(':dao')
}
assume we want our library in the root project in folder called libs, for example I have put xpp3.jar in that folder and I want to reference it inside my projects
1- add flat directory repository :
repositories {
mavenCentral( )
flatDir {
dirs "$rootDir/libs"
}
}
$rootDir is a DSL variable that points to the root project base directory.
and in the dependencies add :
compile 'xpp3:xpp3:1.1.4'
it will now be resolved to the libs directory without the need to complex configuration like maven.
another way to do this is to use the files( ) method without declaring a flatDir repository:
compile files('../libs/commons-fileupload-1.3.1.jar') //[assuming we are in a subproject depedency ]
see the master-proj build.gradle :
ok now the final step is prepare our application for deployment, I have took some time to add the required spring configurations and wire everything together I added few dependencies for junit in the master project build.gradle:
[':bl', ':dao', ':rest'].each { pn ->notice the scope of junit is for test compile only so it won't be packaged with the final war.
project(pn) {
dependencies {
compile 'org.springframework:spring-context:4.1.0.RELEASE'
compile project(':be')
testCompile group: 'junit', name: 'junit', version: '4.+'
testCompile 'org.springframework:spring-test:4.1.0.RELEASE'
}
}
}
to deploy the project run :
gradle build and you will get a war from the rest project, drop that in your server and you're good to go
visit the final project setup here on git hub:
https://github.com/blabadi/hello-world/tree/dev/test-gradle/master-proj
Summary :
Gradle is simple, flexible and really powerful, the only thing took time is boilerplate required for the initial structure which I did manually on purpose to understand the very basics of gradle.
Gradle yet has more interesting features, and what I only covered is mainly the java plugin and maven in gradle, the possibilities are endless, since you are using groovy scripting for the build domain (DSL) which provides easy to change conventions.
refs :
1- http://www.gradle.org/docs/current/userguide/
2- http://spring.io/guides/gs/gradle/
Thursday, September 11, 2014
Using git hub with eclipse (EGit)
I added a new section to clone a repo from the scratch by EGit without using any external tool like Git Bash
Section 1 : Working with existing Repo
Today I'm going to try EGit to integrate a local and remote repository with eclipse
let's start by running eclipse > File > Import :
1 |
2 |
3 |
4 |
5 |
6 |
Then I added a Class called Tester to this project and committed it through git bash ( to test if eclipse will sync if I use git bash)
7 |
8 |
9 |
Until then the project didn't look like it was connected with my local repository, I right-clicked the project in eclipse > Team > share project :
10 |
11 |
After I clicked finish the source control icons in eclipse appeared :
12 |
so eclipse connected well to the local repository without loosing any info as you can see 'Tester.java' is appearing as committed.
Now we will start using EGit, and to do that I added another class 'Tester2' and committed it:
13 |
14 |
Now the file is committed, let's try to push it to the remote repository:
right click the project > Team > push branch
15 |
16 |
17 |
Done! very smooth process with good GUI from eclipse to do basic functionality like adding files and committing then push to remote, lets try more features.
1- branching
create new branch 'dev-adding-feature1':
1 |
2 |
add new Interface : Feature1.java, at first the file won't be tracked by git, to add it to the tracking tree right click the file > Team > Add to Index :
3 |
4 |
but we also want to remove the configuration files from appearing here since they are not to be committed, let's add them to the ignore list:
switch to navigator view ( window -> open view -> navigator )
5 |
6 |
Now let's commit and push to the remote repo:
7 |
8 |
9 |
10 |
this will create a new remote branch on git-hub
11 |
let's do a pull request and merge dev with dev-adding-feature1
13 |
Now let's switch locally to the dev branch instead of dev-adding-feature1 to update our local dev branch with the new changes:
before update (no feature 1) |
go to Team > Remote > Fetch From ..
14 |
17 |
Now the HEAD changes are fetched for all repositories, we will need to merge the remote dev branch with our local dev branch to see the changes:
go to Team > Merge..
from local section, dev branch will be selected, and from Remote section, select origin/dev
Now the new commits (changes) will be available in our local dev branch.
another way to do this is to do a Pull. it will automatically do a fetch then merge.
if a conflict happens we will need to resolve it before merge is possible.
Select Clone URI, then copy the https url and put in your git hub user name and password:
after that the info of the repository will be fetched
Click next and choose the branch that you want to work in, for now I selected master.
after that you will be promted to select a project, in my master I didn't had any so I had to finish the wizard there.
Now because I didn't select the proper branch [ has no projects ] to switch branches in EGit go to to:
Window > Show view > Others : Git > Git Repositories
after that the info of the repository will be fetched
Click next and choose the branch that you want to work in, for now I selected master.
after that you will be promted to select a project, in my master I didn't had any so I had to finish the wizard there.
Now because I didn't select the proper branch [ has no projects ] to switch branches in EGit go to to:
Window > Show view > Others : Git > Git Repositories
Monday, September 8, 2014
Java 8 top features - part 1
First to try the features we are going to use Eclipse Luna because it supports Java 8 out of the box.
So after you download lets open a new project and try some of the new features
1- Lambdas, Functional interfaces, method references
https://gist.github.com/blabadi/b38d4186efe80681b7ef
In this gist, we can see three different ways java 8 allows us to use the three features :
a) Lambdas :
Lambdas are a short way to write a function on this form:
(param1, param2,...) -> { /* function body */ }
there is no need for a return statement if the function body is one statement (i.e. no bracelet brackets are used) examples:
map(name -> name.length()) , can also be written as : map(name-> {return name.length();})
filter(x -> x.length() > 2)
b) Functional interfaces
In Java 8 a functional interface is defined as an interface with exactly one abstract method. [1]
Consumer<String> myPrint = (x) -> {
System.out.println(x);
System.out.println("---");
};
names.stream().filter(x -> {return x.length() > 2;}).forEach(myPrint);
c) method references
instead of writing a new lambda expression each time, if we are going to reuse a function and pass it like lambda expression, java 8 now supports that.
example
names.stream().map(String::length).forEach(System.out::println);
here we ask that for each item in the names collection, get each item length(new collection) then print using the System.out.println method.
this useful for code reuse, a good example for that here :
https://leanpub.com/whatsnewinjava8/read#leanpub-auto-method-references
2- Default methods
this feature allow interfaces to have concrete methods that are not required by implementer to override. this is mainly used to add new methods to old interfaces without breaking all implementing classes. this is similar to have abstract class.
https://gist.github.com/blabadi/926e1b1d54c093dd6e80
https://gist.github.com/blabadi/f0cc8cc40a3598e28fca
https://gist.github.com/blabadi/fa6c472001dc68bbe395
the implementer of stream( ) method added it as default method to the Collection interface[1] in order to no break all other implementers.
3- Streams
It represents a sequence of objects somewhat like the Iterator interface. However, unlike the Iterator, it supports parallel execution.
Streams support multiple helpful methods to manipulate the stream some of them:
1-map : used for projection
2-filter: filter the stream elements based on criteria
3-forEach: iterates over stream elements
these methods are executed lazily [ when they are iterated only ], example of their usage is above.
more about streams here:
https://leanpub.com/whatsnewinjava8/read#leanpub-auto-streams
4- Optional
the Optional class in the java.util package for avoiding null return values (and thusNullPointerException).[1]example:
https://gist.github.com/blabadi/89c4aa03ec868e938939
this gist will output -1:
5- Nashorn
Java 8 supports Javascript out of the box using the Nashorn script engine which means we can execute some java script in the JVM runtime
this also means that our java script can see our Java classes which allows us to create powerful scripts using our exposed Java classes:
https://gist.github.com/blabadi/8314e2616e8ce3e45f79
https://gist.github.com/blabadi/d2c875a59d67a28eae05
https://gist.github.com/blabadi/0f14d19adcbedccc220e
References:
[1] https://leanpub.com/whatsnewinjava8/read
[2] http://howtodoinjava.com/2014/06/09/java-8-optionals-complete-reference/
[3] http://www.oracle.com/technetwork/articles/java/java8-optional-2175753.html
[4] http://winterbe.com/posts/2014/04/05/java8-nashorn-tutorial/
[5] http://blog.jhades.org/java-8-how-to-use-optional/
So after you download lets open a new project and try some of the new features
1- Lambdas, Functional interfaces, method references
https://gist.github.com/blabadi/b38d4186efe80681b7ef
In this gist, we can see three different ways java 8 allows us to use the three features :
a) Lambdas :
Lambdas are a short way to write a function on this form:
(param1, param2,...) -> { /* function body */ }
there is no need for a return statement if the function body is one statement (i.e. no bracelet brackets are used) examples:
map(name -> name.length()) , can also be written as : map(name-> {return name.length();})
filter(x -> x.length() > 2)
b) Functional interfaces
In Java 8 a functional interface is defined as an interface with exactly one abstract method. [1]
Consumer<String> myPrint = (x) -> {
System.out.println(x);
System.out.println("---");
};
names.stream().filter(x -> {return x.length() > 2;}).forEach(myPrint);
c) method references
instead of writing a new lambda expression each time, if we are going to reuse a function and pass it like lambda expression, java 8 now supports that.
example
names.stream().map(String::length).forEach(System.out::println);
here we ask that for each item in the names collection, get each item length(new collection) then print using the System.out.println method.
this useful for code reuse, a good example for that here :
https://leanpub.com/whatsnewinjava8/read#leanpub-auto-method-references
2- Default methods
this feature allow interfaces to have concrete methods that are not required by implementer to override. this is mainly used to add new methods to old interfaces without breaking all implementing classes. this is similar to have abstract class.
https://gist.github.com/blabadi/926e1b1d54c093dd6e80
https://gist.github.com/blabadi/f0cc8cc40a3598e28fca
https://gist.github.com/blabadi/fa6c472001dc68bbe395
the implementer of stream( ) method added it as default method to the Collection interface[1] in order to no break all other implementers.
3- Streams
It represents a sequence of objects somewhat like the Iterator interface. However, unlike the Iterator, it supports parallel execution.
Streams support multiple helpful methods to manipulate the stream some of them:
1-map : used for projection
2-filter: filter the stream elements based on criteria
3-forEach: iterates over stream elements
these methods are executed lazily [ when they are iterated only ], example of their usage is above.
more about streams here:
https://leanpub.com/whatsnewinjava8/read#leanpub-auto-streams
4- Optional
the Optional class in the java.util package for avoiding null return values (and thusNullPointerException).[1]example:
https://gist.github.com/blabadi/89c4aa03ec868e938939
this gist will output -1:
5- Nashorn
Java 8 supports Javascript out of the box using the Nashorn script engine which means we can execute some java script in the JVM runtime
this also means that our java script can see our Java classes which allows us to create powerful scripts using our exposed Java classes:
https://gist.github.com/blabadi/8314e2616e8ce3e45f79
https://gist.github.com/blabadi/d2c875a59d67a28eae05
https://gist.github.com/blabadi/0f14d19adcbedccc220e
References:
[1] https://leanpub.com/whatsnewinjava8/read
[2] http://howtodoinjava.com/2014/06/09/java-8-optionals-complete-reference/
[3] http://www.oracle.com/technetwork/articles/java/java8-optional-2175753.html
[4] http://winterbe.com/posts/2014/04/05/java8-nashorn-tutorial/
[5] http://blog.jhades.org/java-8-how-to-use-optional/
Thursday, September 4, 2014
Git & Git hub 101
step by step on using git hub for first time
create an account on GitHub.com :
1- create a repository
2- create a new branch on the remote repository
if you don't have a read me file, you will not see the branches so click on README
the new branch is to avoid messing the master branch (default branch) this is a good practice to create branches for every feature you work on then merge these branches to master after everything is tested on the branch itself to keep master working and clean.
3- install git bash (http://git-scm.com/downloads) on your machine and create a directory to host the remote repository locally
> mkdir newDir
> git clone repository_name /path/to/localrepo/
create an account on GitHub.com :
1- create a repository
2- create a new branch on the remote repository
if you don't have a read me file, you will not see the branches so click on README
the new branch is to avoid messing the master branch (default branch) this is a good practice to create branches for every feature you work on then merge these branches to master after everything is tested on the branch itself to keep master working and clean.
3- install git bash (http://git-scm.com/downloads) on your machine and create a directory to host the remote repository locally
> mkdir newDir
> git clone repository_name /path/to/localrepo/
in my case it will be
> git clone git@github.com:blabadi/hello-world.git ./newDir
Info : to paste in git bash use ctrl + Insert
from the first try this command failed with me, because I haven't generated an SSH key
to fix that I had to verify my email address to git hub, they sent u an email with link
then follow this guide :
https://help.github.com/articles/generating-ssh-keys
i'll try again now

from the first try this command failed with me, because I haven't generated an SSH key
to fix that I had to verify my email address to git hub, they sent u an email with link
then follow this guide :
https://help.github.com/articles/generating-ssh-keys
i'll try again now
ok done!
4- now let's check what we have in the directory
the .git file is for git not us.. the read me file is the only file we have right now.
ok let's navigate to the repo dir in git bash and see
you will see this : ' ~/blabla/newDir (master)'
this means that we are now in the repo directory and the active branch is master
5- let's switch to dev branch :
6- now let's add a new file and modify read me file
I created a new file called simple.txt inside the repo directory, let's see how can we see that change in gitbash:
this indicates that git has detected a new file but currently it's not tracked and is not included in the commit if we commit. to add it do the following:
Now it's ready to be commited :
now our 'local' dev branch has simple.txt, but if we check online the 'remote' repo doesn't
Git is a distributed Version control system, it has the concept of Remote and Local repositories
Remote repositories act as servers, central points, while local repositories are a clone of the remote repositories but are only used and changed on your machine.
so the work flow for a branch is as following:
create local branch -> do work locally ->commit work -> push to remote branch -> repeat
there are more steps but we will go over them later.
7- let's push our changes to the remote dev branch:
Now check Git hub :
as you can see simple.txt is now there.
try and modify read me file, it goes in the same cycle and steps.
8- let's merge now the remote dev branch with master branch
to do this we need to do a pull request
As you can see in the last image, master now has simple.txt file.
============================================================
Note the below segment is taken from :
https://github.com/GarageGames/Torque2D/wiki/Cloning-the-repo-and-working-with-Git
Important Git concepts:
Repository / Repo : This is the project's source code that resides on github.com's servers. You cannot modify the contents of this repository directly unless you were the one who created it in the first place.
Fork : Forking a project will create a copy of the original repository that you can modify as you please. Forked projects will appear in your own github.com account.
Cloning : this will clone an online repository to your hard drive so you may begin working on your modifications. This local copy is called your local repository.
Branch : A branch is a different version of the same project.
Remote : A remote is simply an alias pointing to an online repository. It is much easier to work with such aliases than typing in the complete URL of online repositories every single time.
Staging Area : Whenever you want to update your online repository (the one appearing in your github.com account), you first need to add your changes to your staging area. Modifying files locally will not automatically update yourstaging area's contents.
Important Git commands
Fetch : git fetch will download the current state (containing updated and newly created branches) of an online repository without modifying your local repository. It places its results in .git/FETCH_HEAD.
Merge : git merge will merge the modifications of another branch into the current working branch.
Pull : git pull is actually a combination of git fetch and git merge. It fetches the information from an online repository's branch and merges it with your local copy.
Add : Whenever you modify a file in your local repository or create a new file, that file will appear as unstaged. Calling git add allows you to specify files to be added to your staging area.
Commit : A commit records a snapshot of your staging area, making it ready to be pushed to an online repository.
Push : git push will take all of your locally committed changes and upload them to a remote repository's branch.
Important Git concepts:
Repository / Repo : This is the project's source code that resides on github.com's servers. You cannot modify the contents of this repository directly unless you were the one who created it in the first place.
Fork : Forking a project will create a copy of the original repository that you can modify as you please. Forked projects will appear in your own github.com account.
Cloning : this will clone an online repository to your hard drive so you may begin working on your modifications. This local copy is called your local repository.
Branch : A branch is a different version of the same project.
Remote : A remote is simply an alias pointing to an online repository. It is much easier to work with such aliases than typing in the complete URL of online repositories every single time.
Staging Area : Whenever you want to update your online repository (the one appearing in your github.com account), you first need to add your changes to your staging area. Modifying files locally will not automatically update yourstaging area's contents.
Important Git commands
Fetch : git fetch will download the current state (containing updated and newly created branches) of an online repository without modifying your local repository. It places its results in .git/FETCH_HEAD.
Merge : git merge will merge the modifications of another branch into the current working branch.
Pull : git pull is actually a combination of git fetch and git merge. It fetches the information from an online repository's branch and merges it with your local copy.
Add : Whenever you modify a file in your local repository or create a new file, that file will appear as unstaged. Calling git add allows you to specify files to be added to your staging area.
Commit : A commit records a snapshot of your staging area, making it ready to be pushed to an online repository.
Push : git push will take all of your locally committed changes and upload them to a remote repository's branch.
Subscribe to:
Posts (Atom)
Istio —simple fast way to start
istio archeticture (source istio.io) I would like to share with you a sample repo to start and help you continue your jou...
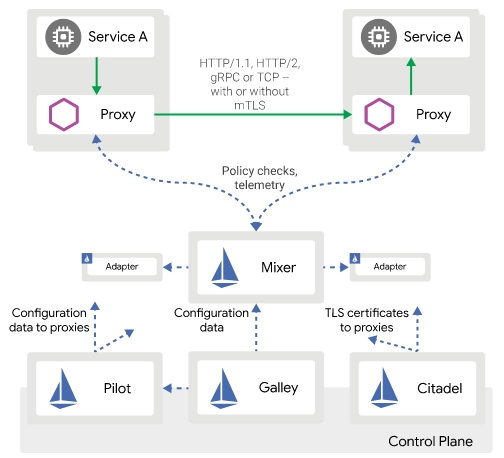
-
So RecyclerView was introduced to replace List view and it's optimized to reuse existing views and so it's faster and more efficient...
-
In the previous part we added the first call to the async api to do search, now we will build on that to call more apis, and to add more c...
-
In this post I'll explain the required work to create a rest API utilizing both spring and hibernate version 4, and the configuration wi...