First to try the features we are going to use Eclipse Luna because it supports Java 8 out of the box.
So after you download lets open a new project and try some of the new features
1- Lambdas, Functional interfaces, method references
https://gist.github.com/blabadi/b38d4186efe80681b7ef
In this gist, we can see three different ways java 8 allows us to use the three features :
a) Lambdas :
Lambdas are a short way to write a function on this form:
(param1, param2,...) -> { /* function body */ }
there is no need for a return statement if the function body is one statement (i.e. no bracelet brackets are used) examples:
map(name -> name.length()) , can also be written as : map(name-> {return name.length();})
filter(x -> x.length() > 2)
b) Functional interfaces
In Java 8 a functional interface is defined as an interface with exactly one abstract method. [1]
Consumer<String> myPrint = (x) -> {
System.out.println(x);
System.out.println("---");
};
names.stream().filter(x -> {return x.length() > 2;}).forEach(myPrint);
c) method references
instead of writing a new lambda expression each time, if we are going to reuse a function and pass it like lambda expression, java 8 now supports that.
example
names.stream().map(String::length).forEach(System.out::println);
here we ask that for each item in the names collection, get each item length(new collection) then print using the System.out.println method.
this useful for code reuse, a good example for that here :
https://leanpub.com/whatsnewinjava8/read#leanpub-auto-method-references
2- Default methods
this feature allow interfaces to have concrete methods that are not required by implementer to override. this is mainly used to add new methods to old interfaces without breaking all implementing classes. this is similar to have abstract class.
https://gist.github.com/blabadi/926e1b1d54c093dd6e80
https://gist.github.com/blabadi/f0cc8cc40a3598e28fca
https://gist.github.com/blabadi/fa6c472001dc68bbe395
the implementer of stream( ) method added it as default method to the Collection interface[1] in order to no break all other implementers.
3- Streams
It represents a sequence of objects somewhat like the Iterator interface. However, unlike the Iterator, it supports parallel execution.
Streams support multiple helpful methods to manipulate the stream some of them:
1-map : used for projection
2-filter: filter the stream elements based on criteria
3-forEach: iterates over stream elements
these methods are executed lazily [ when they are iterated only ], example of their usage is above.
more about streams here:
https://leanpub.com/whatsnewinjava8/read#leanpub-auto-streams
4- Optional
the Optional class in the java.util package for avoiding null return values (and thusNullPointerException).[1]example:
https://gist.github.com/blabadi/89c4aa03ec868e938939
this gist will output -1:
5- Nashorn
Java 8 supports Javascript out of the box using the Nashorn script engine which means we can execute some java script in the JVM runtime
this also means that our java script can see our Java classes which allows us to create powerful scripts using our exposed Java classes:
https://gist.github.com/blabadi/8314e2616e8ce3e45f79
https://gist.github.com/blabadi/d2c875a59d67a28eae05
https://gist.github.com/blabadi/0f14d19adcbedccc220e
References:
[1] https://leanpub.com/whatsnewinjava8/read
[2] http://howtodoinjava.com/2014/06/09/java-8-optionals-complete-reference/
[3] http://www.oracle.com/technetwork/articles/java/java8-optional-2175753.html
[4] http://winterbe.com/posts/2014/04/05/java8-nashorn-tutorial/
[5] http://blog.jhades.org/java-8-how-to-use-optional/
Subscribe to:
Post Comments (Atom)
Istio —simple fast way to start
istio archeticture (source istio.io) I would like to share with you a sample repo to start and help you continue your jou...
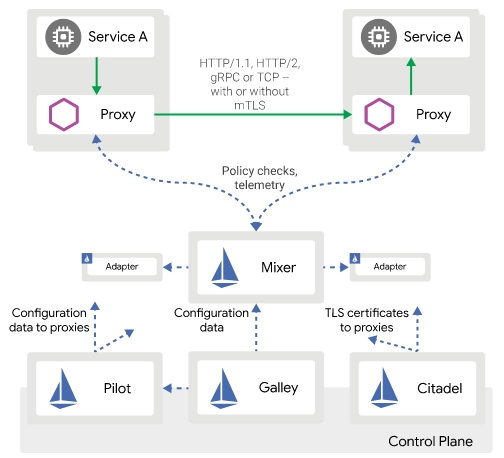
-
I worked before on learning angular 2+, and made a sample functioning app that tracks calories & macros, called it ...
-
So RecyclerView was introduced to replace List view and it's optimized to reuse existing views and so it's faster and more efficient...
-
In the previous part we finished the dashboard read functionality, now we want to add the skeleton for other pages: - Login In this p...
No comments:
Post a Comment
Note: Only a member of this blog may post a comment.